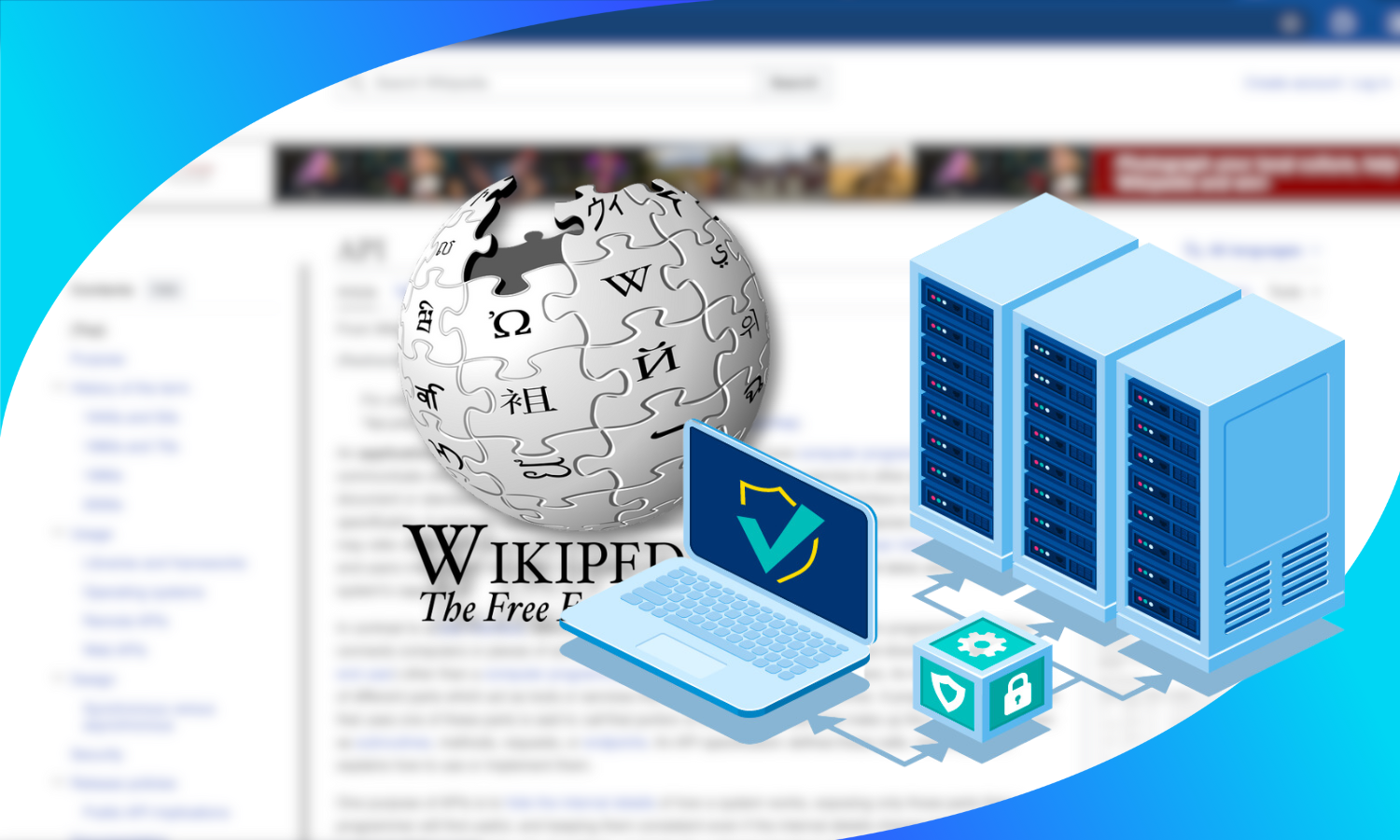
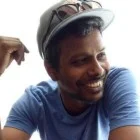
Updated · Feb 11, 2024
Updated · Dec 08, 2023
Muninder Adavelli is a core team member and Digital Growth Strategist at Techjury. With a strong bac... | See full bio
April is a proficient content writer with a knack for research and communication. With a keen eye fo... | See full bio
JSON (Javascript Object Notation) is currently the most popular data transmission format. Almost 50% of the sites worldwide use this format.
It is lightweight and formatted in such a way that is easily readable for humans and machines. JSON is also compatible with a lot of programming languages—including Python.
This article will cover how to read and parse JSON files in Python with easy-to-understand examples. Keep reading to learn more.
Key Takeaways
|
Parsing entails different tools and languages, depending on the format of data that needs to be parsed. Luckily, JSON is a format that is compatible with most tools and programming languages.
When reading or parsing JSON files using Python, the language supports JSON through the pre-installed module known as “json.”
Python calls forth this module like this:
import json |
The json module is capable of converting JSON strings or files into Python dictionaries and vice versa. Here is a table of JSON objects and their corresponding Python data types:
JSON |
Python |
Object |
dict |
Array |
list, tuple |
String |
str |
Number |
int, float |
Null |
None |
True |
True |
False |
False |
For JSON to be readable in Python, its objects must be converted to the corresponding Python data type.
Reading a JSON file in Python always leads to an actual conversion from JSON objects to Python dictionaries. This is done by the functions load and loads (read as load-s or load string) in the json module.
Here is an example of a JSON string:
{ |
To convert this into a Python dict, use the loads() function. Here’s the script:
import json
""" |
The JSON object is successfully converted to a Python dictionary. This is confirmed by the type() function, which printed the <class ‘dict’> result.
The same process is required when parsing a JSON file. To do that, you can employ the load() function.
Say you have a JSON file named exampleuser.json that has the same content as the sample JSON string above.
To read this JSON file, you can:
import json python_dict = {'name': 'John Doe', 'age': 30, 'address': {'street': '123 Main Street', 'city': 'New York', 'state': 'NY'}, 'isPreferredCustomer': True, 'notes': None} |
📝Note: This method is most useful in reading larger JSON files. |
Recall the capability of Python’s json module to convert dictionaries into JSON objects discussed earlier.
In this case, you will make use of the functions dump and dumps (read as dump-s or dump string).
Use the dumps() function to convert Python dictionaries into JSON strings. Here is an example:
import json |
Conversion without data loss is a key advantage of JSON. To make it more readable, you can add the indent argument within the dumps() function.
json_string = json.dumps (python_dict, indent=4) |
This should return a more readable output, like:
{ |
You have to use the dump() function to achieve this. Again, the open() function will open an existing file as indicated.
If it does not exist, it will be automatically created with the help of the write (‘w’) mode. The write mode will also change the content of the file in case it does exist.
Here is a sample script:
import json |
Once done, you now know how to read and parse JSON using a Python module.
👍Helpful Article Besides JSON, you can also parse other formats with Python. Try parsing HTML with RegEx using Python scripts. RegEx is a character sequence widely used in configuring strings of text. |
The JSON module is vital in providing a straightforward way of reading and parsing JSON—with just a few lines of code.
However, this is nowhere near its full capabilities. Make sure to review the json module’s official documentation to dig for more.
One way is to parse JSON with Python’s json module. You can make use of load and loads functions to read JSON objects. You can also specify the key-value pairs.
Use the dump() function from the json module to write objects in a JSON file. You will also need the open() function in write mode to create a file or overwrite an existing one.
It is relatively easier to parse JSON than its alternative, XML. This is from the standpoint of programming languages—such as Python and Javascript. Another reason is JSON is lightweight, making it faster for parsers to work on.
Your email address will not be published.
Updated · Feb 11, 2024
Updated · Feb 11, 2024
Updated · Feb 08, 2024
Updated · Feb 05, 2024